Here's just a quick mention about a Tiny BASIC series that I'm writing over on one of my other blogs. Run BASIC uses a version of Tiny BASIC as an example, so I figure that some Run BASIC users would be interested in seeing what's being done in that blog. Essentially what I'm doing is showing how to extend the Tiny BASIC interpreter. This is of interest for anyone who enjoys BASIC, who is curious about language implementation, and who might find a BASIC interpreter useful as a scripting language for their application written in BASIC.
http://basicprogramming.blogspot.com
See you all there!
Friday, January 8, 2016
Back to business
It's been a long time since I've been blogging on any of my blog pages.
So, now that I've been at it again on the BASIC Programming blog, I posted the following information below when someone inquired about Run BASIC, so it only makes sense to post it here also.
The essential concept of Run BASIC that it is an all-in-one BASIC web application server. You can use it to create a dynamic web site, or to host in-house applications for your business or school, or use it to control your home. The possibilities are pretty much endless.
Here is a link to a white paper about Run BASIC.
http://www.libertybasic.com/RunBASICBreakthrough.pdf
Here is a link to the Run BASIC community forum.
http://runbasic.proboards.com/
So, now that I've been at it again on the BASIC Programming blog, I posted the following information below when someone inquired about Run BASIC, so it only makes sense to post it here also.
The essential concept of Run BASIC that it is an all-in-one BASIC web application server. You can use it to create a dynamic web site, or to host in-house applications for your business or school, or use it to control your home. The possibilities are pretty much endless.
Here is a link to a white paper about Run BASIC.
http://www.libertybasic.com/RunBASICBreakthrough.pdf
Here is a link to the Run BASIC community forum.
http://runbasic.proboards.com/
Tuesday, January 13, 2009
A Simple Table - Lesson 7
Run BASIC provides an easy way to display tabular information in a web app. The TABLE statement takes the data from an array and places it in a table with automatically aligned columns. Here is a simple program that lets you add a row of three items at a time to an array, and then the table grows as each row is added:
In the next lesson we will add some visual decoration to the program.
'simple tableHere is a version for the GOTO averse. ;-)
limit = 200
dim locations$(2, limit)
[displayAll]
cls
if position <= limit then
print "Name: ";
textbox #name, ""
#name setfocus()
print " X:";
textbox #x, ""
print " Y:";
textbox #y, ""
button #add, "Add", [add]
else
print "Limit reached."
end if
if position > 0 then
table #t, locations$()
render #t
end if
wait
[add]
locations$(0, position) = #name contents$()
locations$(1, position) = #x contents$()
locations$(2, position) = #y contents$()
position = position + 1
goto [displayAll]
'simple table
global #name, #x, #y, limit, position
limit = 200
dim locations$(2, limit)
call displayAll
wait
sub displayAll
cls
if position <= limit then
print "Name: ";
textbox #name, ""
#name setfocus()
print " X:";
textbox #x, ""
print " Y:";
textbox #y, ""
button #add, "Add", add
else
print "Limit reached."
end if
if position > 0 then
table #t, locations$()
render #t
end if
end sub
sub add key$
locations$(0, position) = #name contents$()
locations$(1, position) = #x contents$()
locations$(2, position) = #y contents$()
position = position + 1
call displayAll
end sub
In the next lesson we will add some visual decoration to the program.
Monday, January 12, 2009
Module, component, object... Whatsit?
In our last post we discussed how to create a module for reusing code that is common between different programs. You may have noticed that we used the terms module, component, and object interchangeably. We did this so that we could write this post. ;-)
The term object oriented has a bad reputation in some programming circles. People who enjoy structured procedural languages like BASIC, Pascal, C, etc. often find objects challenging to wrap their minds around. Run BASIC incorporates some very simple object oriented ideas and tries to present them in a non-object way. For example you can work with files and graphics without noticing that they are objects in Run BASIC.
Creating a module like we did in the last lesson shows a simple way to leverage objects in BASIC. Nothing fancy really. Just create a separate program and hold onto it in a variable. Then you can call its functions to do things.
That's really all an object is.
Labels:
basic,
component,
library,
modularity,
object oriented,
pascal,
run basic,
web programming
Saturday, January 10, 2009
Making a module - Lesson 6
Many times it can be useful to break a program up into pieces. For example the part of our hypotenuse calculator that draws a right triangle might be useful in more than one program about triangles. Why reimplement it in each one?
Run BASIC permits a program to use one or more others. Let's take the code that draws the right triangle and make a program that can be used as a module (or object) by the hypotenuse calculator program. Here's what that looks like:
Here is how the hypotenuse calculator looks once we modify it to use the new right triangle drawing module:
'hypotenuse calculator with module
global a, b, c, #sideA, #sideB
run "rightTriangle", #drawing
call displayAll
wait
sub displayAll
cls
print "Hypotenuse Calculator"
print
print "Length of side A: ";
textbox #sideA, a
print
print "Length of side B: ";
textbox #sideB, b
print
button #go, "Go!", computeSideC
print
if c > 0 then
print "Length of side C: "; c
#drawing drawRightTriangle(a, b, c)
render #drawing
end if
end sub
sub computeSideC key$
a = #sideA value()
b = #sideB value()
c = sqr(a^2+b^2)
call displayAll
end sub
See how at the beginning of the program we use a RUN statement to start up a copy of the rightTriangle program? Then we assign it to an object variable named #drawing.
Inside our displayAll subroutine we no longer use CALL to access the drawRightTriangle subroutine because it doesn't exist in our program anymore. Instead we invoke the drawRightTriangle() function on the #drawing object using the form:
Whew! That was a mouthful.
Run BASIC permits a program to use one or more others. Let's take the code that draws the right triangle and make a program that can be used as a module (or object) by the hypotenuse calculator program. Here's what that looks like:
'This program is a module for drawingThe idea here is that our hypotenuse calculator program will run this one which will just stop and wait to be called. That's why the first thing in the program is a WAIT statement. Notice also that we convert the SUB into a FUNCTION. This is the format that Run BASIC expects when one program calls another to do some work.
'right triangles.
wait
function drawRightTriangle(a, b, c)
cls
graphic #rightTri, 240, 180
#rightTri place(210, 160)
#rightTri north()
#rightTri go(150)
#rightTri turn(-127)
#rightTri go(250)
#rightTri turn(-143)
#rightTri go(200)
#rightTri box(180, 130)
#rightTri place(215, 80)
#rightTri "\"; a
#rightTri place(100, 170)
#rightTri "\"; b
#rightTri place(90, 80)
#rightTri "\"; c
render #rightTri
end function
Here is how the hypotenuse calculator looks once we modify it to use the new right triangle drawing module:
'hypotenuse calculator with module
global a, b, c, #sideA, #sideB
run "rightTriangle", #drawing
call displayAll
wait
sub displayAll
cls
print "Hypotenuse Calculator"
print "Length of side A: ";
textbox #sideA, a
print "Length of side B: ";
textbox #sideB, b
button #go, "Go!", computeSideC
if c > 0 then
print "Length of side C: "; c
#drawing drawRightTriangle(a, b, c)
render #drawing
end if
end sub
sub computeSideC key$
a = #sideA value()
b = #sideB value()
c = sqr(a^2+b^2)
call displayAll
end sub
See how at the beginning of the program we use a RUN statement to start up a copy of the rightTriangle program? Then we assign it to an object variable named #drawing.
Inside our displayAll subroutine we no longer use CALL to access the drawRightTriangle subroutine because it doesn't exist in our program anymore. Instead we invoke the drawRightTriangle() function on the #drawing object using the form:
#drawing drawRightTriangle(a, b, c)Then once the drawRightTriangle() function is finished we render the #drawing object like so:
render #drawingWait a minute... you say. We already have a RENDER statement in the rightTriangle program. Why do we need another? Good question. Any time you RUN a program from within another and you want the newly RUN program to appear you must render it. So in this case you're not rendering the GRAPHIC object but the whole rightTriangle program is being rendered as part of the page. The RENDER statement in the rightTriangle program just renders the GRAPHIC. So both RENDER statements are needed.
Whew! That was a mouthful.
Labels:
basic,
component,
library,
module,
object oriented,
oop,
run basic,
web programming
Wednesday, January 7, 2009
Turtle graphics - Lesson 5
Let's draw some graphics that we can use with our hypotenuse calculator. Let's draw one of those standard right triangles and then draw values for each side. To do this let's use turtle graphics (here's a Wikipedia entry for turtle graphics). The Run BASIC graphic object has a turtle which you can command by telling it to turn and go on the graphic area. As you command it to go and turn it draws lines showing where it's been.
Examine the drawRightTriangle subroutine to see how we draw the triangle, the little box at the 90 degree corner, and the lengths of each side.
Examine the drawRightTriangle subroutine to see how we draw the triangle, the little box at the 90 degree corner, and the lengths of each side.
'hypotenuse calculatorHere's what the drawing looks like:
global a, b, c, #sideA, #sideB
call displayAll
wait
sub displayAll
cls
print "Hypotenuse Calculator"
print "Length of side A: ";
textbox #sideA, a
print "Length of side B: ";
textbox #sideB, b
button #go, "Go!", computeSideC
if c > 0 then
print "Length of side C: "; c
call drawRightTriangle a, b, c
end if
end sub
sub computeSideC key$
a = #sideA value()
b = #sideB value()
c = sqr(a^2+b^2)
call displayAll
end sub
sub drawRightTriangle a, b, c
graphic #rightTri, 240, 180
#rightTri place(210, 160)
#rightTri north()
#rightTri go(150)
#rightTri turn(-127)
#rightTri go(250)
#rightTri turn(-143)
#rightTri go(200)
#rightTri box(180, 130)
#rightTri place(215, 80)
#rightTri "\"; a
#rightTri place(100, 170)
#rightTri "\"; b
#rightTri place(90, 80)
#rightTri "\"; c
render #rightTri
end sub
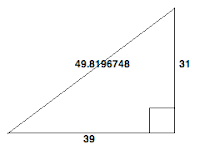
Labels:
basic,
geometry,
graphics,
turtle graphics,
web programming
Monday, January 5, 2009
Drawing Graphics - Lesson 4
Drawing graphics is a powerful and fun feature of programming languages, and Run BASIC makes it easy to do this in a web application.
To do this we use the GRAPHIC statement to create a graphical object. Then we call methods on that object. The term 'method' is another way of saying a function that is tied to a kind of object. Once we've drawn something we can tell Run BASIC to embed the graphic into the web page by using the RENDER statement.
Here is a very simple Run BASIC program that draws a graphic.
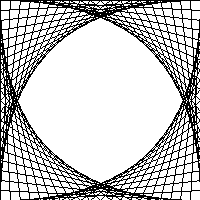
Next lesson we will add a graphical drawing to our hypotenuse calculator.
To do this we use the GRAPHIC statement to create a graphical object. Then we call methods on that object. The term 'method' is another way of saying a function that is tied to a kind of object. Once we've drawn something we can tell Run BASIC to embed the graphic into the web page by using the RENDER statement.
Here is a very simple Run BASIC program that draws a graphic.
'draw a graphic 200 by 200
graphic #pattern, 200, 200
for x = 0 to 200 step 10
#pattern line(x, 0, 0, 200-x)
#pattern line(0, x, x, 200)
#pattern line(x, 0, 200, x)
#pattern line(x, 200, 200, 200-x)
next x
render #pattern
end
Here is what the drawn graphic looks like!
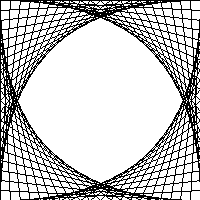
Next lesson we will add a graphical drawing to our hypotenuse calculator.
Labels:
graphics,
logo,
qbasic,
runbasic,
turtle graphics,
web programming
Sunday, January 4, 2009
Using Subroutines - Lesson 3
The first two lessons show how to write old style BASIC programs which use GOTO. Let's show how to create the hypotenuse calculator in a more structured way using scoped subroutines. Here is the code:
If you compare this example to the one for lesson 2, you'll see most of the code is the same.
'hypotenuse calculator
global a, b, c, #sideA, #sideB
call displayAll
wait
sub displayAll
cls
print "Hypotenuse Calculator"
print "Length of side A: ";
textbox #sideA, a
print "Length of side B: ";
textbox #sideB, b
button #go, "Go!", computeSideC
if c > 0 then print "Length of side C: "; c
end sub
sub computeSideC key$
a = #sideA value()
b = #sideB value()
c = sqr(a^2+b^2)
call displayAll
end sub
If you compare this example to the one for lesson 2, you'll see most of the code is the same.
- The first few lines of code kick the program off by calling the displayAll subroutine.
- The displayAll subroutine adds a button which calls the computeSideC subroutine when it is clicked.
- The computeSideC subroutine does some math and then calls the displayAll subroutine, forcing the web page to update.
Labels:
basic,
goto,
lessons,
subroutines,
web programming
Friday, January 2, 2009
Creating a Web Form - Lesson 2
Let's take the simple interactive hypotenuse calculator from the previous post and create a version of it that has a couple of fields and a button to do the computation:
Then the BUTTON statement adds a button that will use the code at the [go] label to compute the length of the C side.
Notice the IF THEN statement after the BUTTON statment. If the length of C is greater than zero then that means we have computed at least once, so print the result on the web page.
[displayAll]The first part of the program clears the web page using the CLS statement. Then it adds labels and entry fields using the PRINT and TEXTBOX statements.
cls
print "Hypotenuse Calculator"
print "Length of side A: ";
textbox #sideA, a
print "Length of side B: ";
textbox #sideB, b
button #go, "Go!", [go]
if c > 0 then print "Length of side C: "; c
wait
[go]
a = #sideA value()
b = #sideB value()
c = sqr(a^2+b^2)
goto [displayAll]
Then the BUTTON statement adds a button that will use the code at the [go] label to compute the length of the C side.
Notice the IF THEN statement after the BUTTON statment. If the length of C is greater than zero then that means we have computed at least once, so print the result on the web page.
Thursday, January 1, 2009
A Simple Interactive Web Application - Lesson 1
One thing that Run BASIC does better than most other web programming systems is interactive applications where a program asks the user for a piece of information at a time and produces answers as you go. This is the way a lot of non-web applications work but it is hard to create these kinds of apps using conventional web programming.
Here is Run BASIC source code for a simple web application that computes the length of a hypotenuse for a right triangle if the length of the other two sides are known.
If you're completely new to web programming you may not realize that the above code doesn't look like code for a web app. In fact it looks very much like code for a QBasic program. If you're an experienced web programmer you may be scratching your head because it looks too simplistic to be a web program, but it is a web program.
Run BASIC does its best to hide the hard stuff that most web programming systems force the programmer to deal with. If you really must use some special web development technique Run BASIC provides ways to do that too and we'll cover some of these in later posts. Most of the time it isn't necessary because Run BASIC does the heavy lifting for you.
Here is Run BASIC source code for a simple web application that computes the length of a hypotenuse for a right triangle if the length of the other two sides are known.
[startHere]
print "Hypotenuse Calculator"
input "Length of side A"; a
input "Length of side B"; b
c = sqr(a^2+b^2)
print "Length of hypotenuse (side C) = "; c
input "Compute another (Y/N)"; yn$
if yn$ = "Y" or yn$ = "y" then
cls
goto [startHere]
end if
print "Goodbye."
end
If you're completely new to web programming you may not realize that the above code doesn't look like code for a web app. In fact it looks very much like code for a QBasic program. If you're an experienced web programmer you may be scratching your head because it looks too simplistic to be a web program, but it is a web program.
Run BASIC does its best to hide the hard stuff that most web programming systems force the programmer to deal with. If you really must use some special web development technique Run BASIC provides ways to do that too and we'll cover some of these in later posts. Most of the time it isn't necessary because Run BASIC does the heavy lifting for you.
Labels:
basic,
learn,
qbasic,
run basic,
web programming
Saturday, December 27, 2008
Web Programming without Web Programming
This blog will include short lessons that show how to do many different things in Run BASIC, a web programming so easy that it's fair to call it "Web Programming without Web Programming." I call it this because web programming is hard, but Run BASIC is not hard.
Labels:
basic,
learn,
lessons,
programming,
run basic,
software development,
web,
web development,
web programming
Subscribe to:
Posts (Atom)